In intermediate-level interviews, candidates are frequently asked to ‘combine‘, ‘merge‘, ‘join‘, or ‘concatenate‘ two lists in C# programming. Alternatively, interviewers might rephrase the query to ‘merge two sorted lists in C#‘. Regardless of the exact wording, the expected response remains consistent.
FREE : If you’re a new visitor preparing for an interview and need help or want to request a new question, you can use the form on the right side to ask your question. It’s free and doesn’t require signing in. Alternatively, you can ask in our Quora space, which is also free, and you’ll receive a response within 24 hours. Go ahead and check it out! do check, TCS Interview Question – who won the election, Solve Pass The Pillow Problem in C# With 2 Easy Algorithms, Inheritance in OOPs: Ultimate Guide for Coding Interviews, Master Abstract Factory Design Pattern for Programming Interviews with 5 easy steps ,Mastering Object-Oriented Programming in C++.
If you are Unity Developer and instructed to learn ECS and DOTs check my new blog. where I will convert Non-ECS unity project to ECS project.
Table of Contents
- Understand Question Merge Two Lists
- Understand Logic to Join Two Lists in C#
- Program to Merge Two Lists in C#
- Program to Merge Two List in C# Using Linq
- Program to Concat Two Array in C# using Linq
- Summarizing Merge Two Lists in C# Program
- FAQs
- What is the best way to merge two lists in C#?
- How can I merge two sorted lists in C# without using LINQ?
- Can I use LINQ to merge two lists in C#?
- What is the difference between merging and concatenating two lists in C#?
- How do I handle lists of different lengths when merging in C#?
- What is the time complexity of merging two lists in C#?
- Is it possible to merge two lists in C# using a single loop?
- How do I merge two lists in C# if they contain duplicate elements?
- Can I merge two lists of different data types in C#?
- What happens if both lists are empty when trying to merge them in C#?
Understand Question Merge Two Lists
As the title suggests, the interviewer will provide two lists or arrays to perform a join. Sometimes, they may ask to merge two sorted lists or arrays. In both cases, the logic remains the same, so don’t be discouraged.
Let’s comprehend the question through an example. Suppose we have two lists: the first named listA and the second named listB, containing values like this: listA = {1,2,3,4} and listB = {5,6,7,8,9,10}. Your output response would be outputList = {1,2,3,4,5,6,7,8,9,10}. I trust you grasp the objective of merging the two lists.
Do not be afraid of the question; we will cover both methods to concatenate two lists in C# without LINQ and with LINQ.
Understand Logic to Join Two Lists in C#
Let’s comprehend the logic behind joining two sorted lists or merging two lists in C#. In this program, we have two lists, A and B, both of which are already sorted. Let’s assume that A = {2, 4, 6} and B = {1, 3, 5, 7, 9, 10}. Now, you have two choices to merge those lists. First, combine the lists into a single list and then sort it. Second approach is to first sort the lists by each element and simultaneously add the sorted elements to the list.
In this post, I am going to follow the second approach. To begin, create a loop to fetch each element from both lists. During this process, compare the elements from each list to determine which one is smaller. By doing so, both lists will merge into a single list.
In some cases, both lists have different element counts, so you have to add the remaining elements from each list using a loop.
It’s quite confusing, isn’t it? Don’t worry. In the next section, I’ll implement this logic in code so you can understand the rest of the process there.
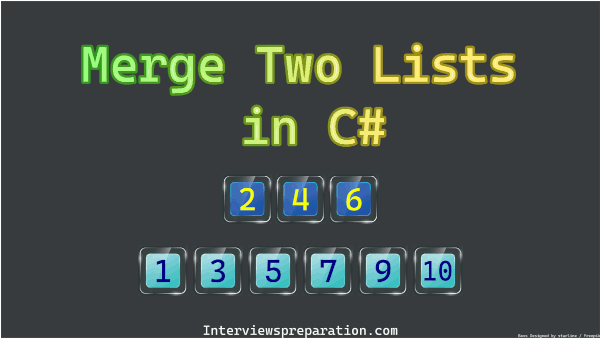
Check above c# merge two lists illustration gif to understand how c# combine two lists works.
Program to Merge Two Lists in C#
Let’s divide it into small steps to implement the logic clearly in the program. If you are a new visitor and not familiar with our work, I suggest checking out our Pattern, Pyramid, Number, and Array programs.
Step 1
The first step is simple: create the required variables according to the logic you have understood. In our case, I’m going to add an integer list named ‘outputList’ and two integer variables to use for retrieving elements from the list.
static List<int> MergeSortedLists(List<int> listA, List<int> listB)
{
List<int> outputList = new List<int>();
int i = 0, j = 0;
return outputList;
}
Step 2
The second step is to create a loop to fetch an element from both lists, compare the elements using less than or equal to to determine the smaller element. Then, add that smaller element to the ‘outputList’ list and increment the respective integer variable (‘i’ and ‘j’ in our case). Additionally, add the end iteration logic, which checks that our integer variables must be smaller than the list count.
static List<int> MergeSortedLists(List<int> listA, List<int> listB)
{
List<int> outputList = new List<int>();
int i = 0, j = 0;
while (i < listA.Count && j < listB.Count)
{
if (listA[i] < listB[j])
{
outputList.Add(listA[i]);
i++;
}
else
{
outputList.Add(listB[j]);
j++;
}
}
return outputList;
}
Step 3
By following these steps, you’ll notice that joining two lists is 80% complete. This is because if both lists have the same number of elements, the last element from one list will be missing. Alternatively, if the lists have different numbers of elements, the elements from the list with the greater count will be missing. To solve this issue, we use another loop to add those missing elements.
static List<int> MergeSortedLists(List<int> listA, List<int> listB)
{
List<int> outputList = new List<int>();
int i = 0, j = 0;
while (i < listA.Count && j < listB.Count)
{
if (listA[i] < listB[j])
{
outputList.Add(listA[i]);
i++;
}
else
{
outputList.Add(listB[j]);
j++;
}
}
while (i < listA.Count)
{
outputList.Add(listA[i]);
i++;
}
while (j < listB.Count)
{
outputList.Add(listB[j]);
j++;
}
return outputList;
}
Step 4
The final step is to call this method and provide two sorted lists to obtain a single sorted list.
static void Main(string[] args)
{
List<int> listA = new List<int> { 2, 4, 6 };
List<int> listB = new List<int> { 1, 3, 5, 7, 9, 10 };
List<int> mergedList = MergeSortedLists(listA, listB);
foreach (var item in mergedList)
{
Console.Write(item + " ");
}
}
Program to Merge Two List in C# Using Linq
This section has been updated following requests from some of our developers to include a LINQ method for merging two lists into a single list.
I hope you enjoyed the previous section where we discussed concatenating two lists in C# without using LINQ. In the following section, we will explore how to achieve this with LINQ. Before we proceed, I would like to highlight that since we will be using LINQ, you need to include the System.Linq namespace to access the LINQ extension methods.
Step 1
To join two lists, we require a specific method to accomplish the merging process. LINQ provides the Concat method to achieve this. Please refer to the official documentation for details on the LINQ syntax and the generic return types.
Let’s proceed with the example of comcat two list using linq in c#. I assume you already have a basic structure for the programme where we have two lists. In my case, I have two lists named list1 and list2.
using System;
using System.Collections.Generic;
using System.Linq;
class Program
{
static void Main()
{
List<int> list1 = new List<int> { 1, 2, 3 };
List<int> list2 = new List<int> { 4, 5, 6 };
}
}
Step 2
Let’s implement the LINQ Concat method to merge two lists. Please pay close attention to the syntax, as it is often overlooked during interviews.
The syntax is quite simple. First, use your first list, then access the LINQ extension method by using the dot (.) operator and typing Concat. In the parentheses, pass your second list as the parameter of the Concat method. After that, use the dot operator again to convert the result to a list by calling the ToList() method, also provided by LINQ. I hope this explanation is clear, but if not, refer to the code below.
using System;
using System.Collections.Generic;
using System.Linq;
class Program
{
static void Main()
{
List<int> list1 = new List<int> { 1, 2, 3 };
List<int> list2 = new List<int> { 4, 5, 6 };
// Merge lists using LINQ
var mergedList = list1.Concat(list2).ToList();
Console.WriteLine("Merged List:");
foreach (var item in mergedList)
{
Console.Write(item + " ");
}
}
}
Program to Concat Two Array in C# using Linq
I don’t want to stretch this out by repeating theory unnecessarily, so let’s keep it simple. LINQ is designed to provide generic programming, so even if you follow the same steps with the list concatenation process, you’ll get the desired output. The only condition is to use ToArray instead of ToList. Let’s check the code to help clarify this.
using System;
using System.Linq;
class Program
{
static void Main()
{
int[] array1 = { 1, 2, 3 };
int[] array2 = { 4, 5, 6 };
// Merge arrays using LINQ
var mergedArray = array1.Concat(array2).ToArray();
Console.WriteLine("Merged Array:");
foreach (var item in mergedArray)
{
Console.Write(item + " ");
}
}
}
Summarizing Merge Two Lists in C# Program
In summary, merging, joining, combining, or concatenating two lists in C# involves fetching elements from both lists, comparing them, and adding the smaller elements to a new list. This process ensures the creation of a single sorted list, thereby achieving the desired outcome efficiently.
By following these steps, you can effectively concatenate two lists in C#.
FAQs
What is the best way to merge two lists in C#?
The best way to merge two lists in C# is to iterate through both lists simultaneously, comparing elements and adding the smaller element to a new list until all elements from both lists are added.
How can I merge two sorted lists in C# without using LINQ?
To merge two sorted lists in C# without using LINQ, you can create a new list and use two pointers to iterate through both lists. Compare the current elements and add the smaller one to the new list, incrementing the pointer accordingly. Finally, add any remaining elements from both lists.
Can I use LINQ to merge two lists in C#?
Yes, you can use LINQ to merge two lists in C#. The Concat
method allows you to combine two lists, and the OrderBy
method can be used to sort the merged list.
What is the difference between merging and concatenating two lists in C#?
Merging typically refers to combining two sorted lists into a single sorted list. Concatenating means joining two lists end-to-end without necessarily sorting the resulting list.
How do I handle lists of different lengths when merging in C#?
When merging lists of different lengths, iterate through both lists, adding the smaller element to the new list. After one list is exhausted, add the remaining elements from the other list to the new list.
What is the time complexity of merging two lists in C#?
The time complexity of merging two lists in C# is O(n + m), where n and m are the lengths of the two lists. This is because each element from both lists is processed exactly once.
Is it possible to merge two lists in C# using a single loop?
No, you need at least two loops: one for comparing and adding elements from both lists, and additional loops to add remaining elements after one list is exhausted.
How do I merge two lists in C# if they contain duplicate elements?
You can merge two lists containing duplicate elements by using the same method of comparison and addition, treating duplicates like any other elements.
Can I merge two lists of different data types in C#?
To merge two lists of different data types, you would need to use a common base type or convert the lists to a common type before merging. Generics can also be used for more flexibility.
What happens if both lists are empty when trying to merge them in C#?
If both lists are empty, the resulting merged list will also be empty, as there are no elements to combine.