Merge two JSON objects or files is an intermediate-level interview question, and sometimes interviewers may phrase it as ‘join,’ ‘combine,’ ‘concatenate,’ or ‘multiply’ two JSON objects. These terms might be used interchangeably to inquire about the process of merging JSON data.
Welcome back, visitors! In this article, I’m going to delve into the logic behind merging JSON objects. Join me as we explore the solution to concatenating two JSON strings in C#. I am going to cover how to join two json objects
FREE : If you’re a new visitor preparing for an interview and need help or want to request a new question, you can use the form on the right side to ask your question. It’s free and doesn’t require signing in. Alternatively, you can ask in our Quora space, which is also free, and you’ll receive a response within 24 hours. Go ahead and check it out! do check. Finding Maximum Profit in Item Sales Based on Categories and Prices, Solve Pass The Pillow Problem in C# With 2 Easy Algorithms , Inheritance in OOPs: Ultimate Guide for Coding Interviews ,Master Abstract Factory Design Pattern for Programming Interviews with 5 easy steps ,Mastering Object-Oriented Programming in C++.
If you are Unity Developer and instructed to learn ECS and DOTs check my new blog. where I will convert Non-ECS unity project to ECS project.
Table of Contents
- What Is Json ?
- How To Join Json Files Or Objects
- Program To Merge Two Json Or Files
- Program To Merge Multiple Json Objects or Files
- Summarizing Merge Two Json Or Multiple Json
- FAQs
- What is JSON?
- Why is merging JSON objects important?
- How do you merge two JSON objects in C#?
- What library is commonly used for handling JSON in C#?
- Can you merge more than two JSON objects at once?
- What are the basic steps to merge two JSON files in C#?
- What is the role of dictionaries in merging JSON objects in C#?
- Is there an alternative to using dictionaries for merging JSON in C#?
- What should you consider when merging JSON objects with conflicting keys?
- How can you validate the merged JSON object to ensure correctness?
What Is Json ?
It is crucial to understand what JSON is because without knowing its structure, you cannot create any logic to manipulate JSON data.
JSON stands for JavaScript Object Notation, which is a text format that humans can read. Below is an example of JSON:
{
"name": "MergeTwoJson",
"id": 2
}
How To Join Json Files Or Objects
Let’s delve into the heart of this article: Concatenating two JSON objects. I hope you are aware of what JSON is and how it works.
To combine multiple JSON objects, I’m going to use C#’s Dictionary. Using this, we can accomplish our merging within 3 steps.
We will store both JSON data into a dictionary and then use any JSON object converter library to create serialized versions of both JSON files.
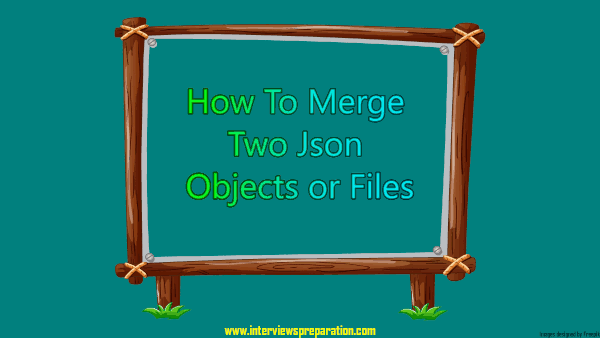
Program To Merge Two Json Or Files
Allows’s initiate our implementation to merge two JSON objects or files.
Step 1
Firstly, we need to have two JSON objects or files. In our case, I’m going to create two JSON files and use the following JSON data to store in them.
// fileone.txt
{
"name": "interviewspreparation.com",
"id": 1
}
// filetwo.txt
{
"name": "MergeTwoJson",
"id": 2
}
Step 2
Now that we have two JSON objects, let’s write code to read both JSON files and store their contents in two JSON objects.
static void Main(string[] args)
{
var jsonOne JsonConvert.DeserializeObject<Dictionary<string, object>>(File.ReadAllText(PathFileOne));
var jsonTwo JsonConvert.DeserializeObject<Dictionary<string, object>>(File.ReadAllText(PathFileTwo));
}
As discussed in the above section, I’m going to use a Dictionary to combine two JSON objects. Therefore, I have used the ‘JsonConvert.DeserializeObject<Dictionary<string, object>>‘ code.
As well, I have used the Newtonsoft.Json library to deserialize JSON strings to a dictionary.
Step 3
Finally, I’m going to use those two objects to serialize them and create a JSON string. This will be our output, concatenating two JSON objects.
static void Main(string[] args)
{
var jsonOne = JsonConvert.DeserializeObject<Dictionary<string, object>>(File.ReadAllText(PathFileOne));
var jsonTwo = JsonConvert.DeserializeObject<Dictionary<string, object>>(File.ReadAllText(PathFileTwo));
var jsonArray = new List<Dictionary<string, object>> { jsonOne, jsonTwo };
string mergedJson = JsonConvert.SerializeObject(jsonArray);
Console.WriteLine(mergedJson);
}
Alternatively, you can use an array to fetch your joined JSON objects.
static void Main(string[] args)
{
var jsonOne = JsonConvert.DeserializeObject<Dictionary<string, object>>(File.ReadAllText(PathFileOne));
var jsonTwo = JsonConvert.DeserializeObject<Dictionary<string, object>>(File.ReadAllText(PathFileTwo));
var jsonArray = new List<Dictionary<string, object>> { jsonOne, jsonTwo };
string mergedJson = JsonConvert.SerializeObject(jsonArray);
Console.WriteLine(mergedJson);
Profile[] profiles = JsonConvert.DeserializeObject<Profile[]>(mergedJson);
foreach (var item in profiles)
{
Console.WriteLine(item.Id + " ___ " + item.Name);
}
}
Program To Merge Multiple Json Objects or Files
Merging more than two JSON objects is not a big deal when you use the above code. You just need to change the two static objects to a loop and store each JSON into a list of dictionary, then serialize it. Check the code below to understand more.
static void Main(string[] args)
{
List<Dictionary<string, object>> jsonArray = new List<Dictionary<string, object>>();
string[] Paths = { PathFileOne, PathFileTwo, PathFileThree};
foreach (string path in Paths)
{
var json = JsonConvert.DeserializeObject<Dictionary<string, object>>(File.ReadAllText(path));
jsonArray.Add(json);
}
string mergedJson = JsonConvert.SerializeObject(jsonArray);
Console.WriteLine(mergedJson);
Profile[] profiles = JsonConvert.DeserializeObject<Profile[]>(mergedJson);
foreach (var item in profiles)
{
Console.WriteLine(item.Id + " ___ " + item.Name);
}
}
Summarizing Merge Two Json Or Multiple Json
Understanding JSON and Its Importance JSON, short for JavaScript Object Notation, serves as a lightweight data-interchange format widely used in modern programming. Its human-readable format and simplicity make it an ideal choice for data representation and exchange in various applications.
Merging JSON Objects in C# Using Dictionaries In C#, dictionaries offer a convenient approach for merging JSON objects. By deserializing JSON strings into dictionaries and merging them, developers can efficiently concatenate JSON data. Libraries like Newtonsoft.Json facilitate this process, providing robust serialization and deserialization capabilities.
Extending Merging Logic for Multiple JSON Objects To merge more than two JSON objects, developers can extend the merging logic by iterating through the objects dynamically. This scalable approach involves storing JSON data in dictionaries iteratively before serialization. By implementing this method, developers ensure flexibility and reusability in handling multiple JSON objects efficiently.
FAQs
What is JSON?
JSON, or JavaScript Object Notation, is a text format for structuring data that is easily readable by humans and used for data interchange.
Why is merging JSON objects important?
Merging JSON objects is crucial in various applications where combining data from multiple sources is required, such as aggregating API responses or merging configuration files.
How do you merge two JSON objects in C#?
In C#, you can merge two JSON objects by deserializing them into dictionaries using the Newtonsoft.Json library, then combining these dictionaries and serializing the result back to a JSON string.
What library is commonly used for handling JSON in C#?
The Newtonsoft.Json library, also known as Json.NET, is commonly used for serializing and deserializing JSON data in C#.
Can you merge more than two JSON objects at once?
Yes, you can merge multiple JSON objects by iterating through each JSON file, deserializing them into dictionaries, adding them to a list, and then serializing the list into a JSON string.
What are the basic steps to merge two JSON files in C#?
The basic steps include: reading the JSON files, deserializing them into dictionaries, merging the dictionaries, and serializing the combined dictionary back into a JSON string.
What is the role of dictionaries in merging JSON objects in C#?
Dictionaries are used to store key-value pairs from JSON objects, allowing easy merging by combining entries from multiple dictionaries before converting back to a JSON format.
Is there an alternative to using dictionaries for merging JSON in C#?
While dictionaries are a common method, you can also use other data structures like arrays or custom objects, depending on the specific requirements of your JSON structure.
What should you consider when merging JSON objects with conflicting keys?
When merging JSON objects with conflicting keys, you need to decide on a strategy for handling conflicts, such as overriding values, combining them into lists, or applying custom logic.
How can you validate the merged JSON object to ensure correctness?
After merging, you can validate the JSON object by deserializing it and checking the structure and values against expected formats or using JSON schema validation tools.