Finding the largest sum subarray is a intermediate problem in coding interviews. In this guide, we’ll explore how to locate the maximum sum of a continuous subarray using Kadane’s Algorithm. Don’t worry if this sounds complex at first—we’ll break it down step by step.
In programming, one common problem is to find the largest sum of a subarray within a given array of integers. This can be efficiently solved using Kadane’s Algorithm. Let’s delve into how to locate the maximum sum of a continuous subarray with this approach.
FREE Offer: If you’re a new visitor preparing for an interview and need help, you can use the form on the right side to ask your question. It’s free and doesn’t require signing in. Alternatively, you can ask in our Quora space, which is also free, and you’ll receive a response within 24 hours. Go ahead and check it out! Don’t miss our top tips on Understanding object oriented programming oop in cpp , reverse a linked list recursively and Function Overloading in C++. Your dream job is just a click away!
Table of Contents
- What is a Subarray?
- Understanding the Largest Sum Subarray Problem
- Why Kadane’s Algorithm ?
- How Kadane’s Algorithm Works ?
- Implement Kadane’s Algorithm in Python
- Detailed Explanation of Kadane’s Algorithm
- Summarizing Kadane’s Algorithm in C++ and Python
- FAQs
- What is Kadane’s Algorithm and why is it used?
- How does Kadane’s Algorithm handle negative numbers in the array?
- Can Kadane’s Algorithm be implemented in Python?
- Why is Kadane’s Algorithm considered a greedy algorithm?
- What is the initial step in Kadane’s Algorithm?
- How do you iterate through the array in Kadane’s Algorithm?
- How does Kadane’s Algorithm ensure it finds the largest sum?
- Can Kadane’s Algorithm be implemented in C++?
- What is a subarray in the context of Kadane’s Algorithm?
What is a Subarray?
Don’t be intimidated by the term “subarray”—we’ll make it simple. A subarray is a sequence of elements from an array that are right next to each other.
For example, in the array [1, 2, 3, 4, 5], the subarray [2, 3, 4] includes elements that are in consecutive positions. Think of it as selecting a continuous chunk out of a longer list. Pretty straightforward, isn’t it?
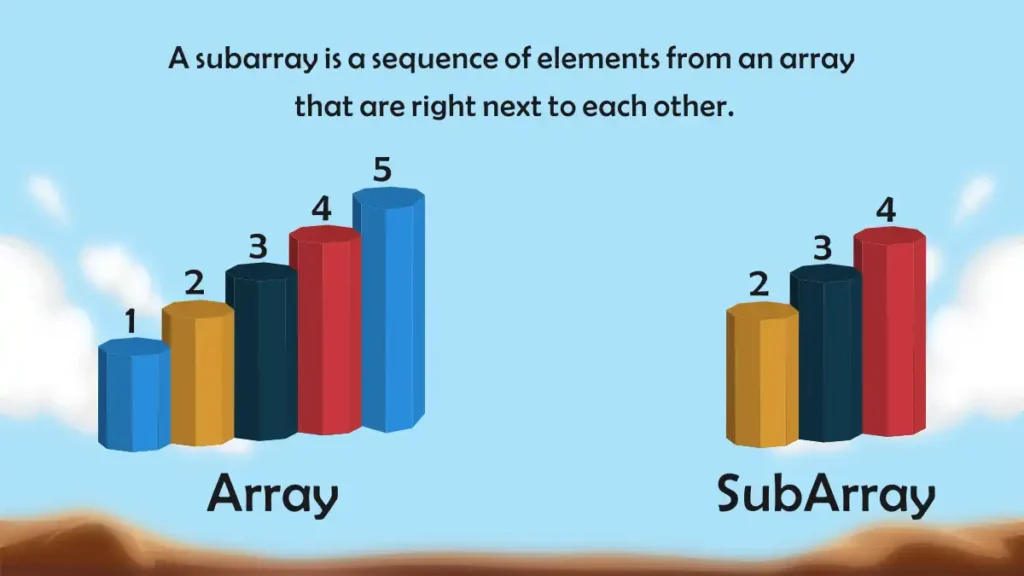
Understanding the Largest Sum Subarray Problem
Imagine you have an array of numbers, like [-2, 1, -3, 4, -1, 2, 1, -5, 4]. Your task is to find a contiguous subarray (a subarray with consecutive elements) that has the largest sum. For this example, the subarray [4, -1, 2, 1] has the largest sum, which is 6.
I hope you now understand what a subarray is and how we locate the maximum sum of a subarray. If not, don’t worry—we’ll cover it in the upcoming sections. Next, we’ll delve into Kadane’s Algorithm, explain why it works so well for solving the maximum sum subarray problem, and provide step-by-step examples. Stay with us!
Why Kadane’s Algorithm ?
As a programmer, you might be wondering, why Kadane’s Algorithm? Well, let me tell you a story. When I was searching for a better approach to solve the continuous subarray problem, I discovered Kadane’s Algorithm.
This technique uses dynamic programming to efficiently find the maximum sum of a continuous subarray. It works in linear time, making it perfect for handling large datasets. The main idea is to iterate through the array, keeping track of both the maximum sum encountered so far and the current subarray sum. Let’s explore next section how it works!
How Kadane’s Algorithm Works ?
Initialize Variables: Start with two variables, max_so_far and max_ending_here, both set to the first element of the array. The max_so_far keeps the record of the maximum sum found, and max_ending_here keeps the sum of the current subarray.
Iterate through the Array: For each element in the array, update max_ending_here to be the maximum of the current element itself or the current element plus max_ending_here. Update max_so_far to be the maximum of max_so_far and max_ending_here.
Return the Result: After processing all elements, max_so_far will contain the largest sum of a subarray.
If you still find it difficult, then refer below table of each iteration of How Kadane’s Algorithm Works. In that illustration, you can see how Kadane’s Algorithm works to solve the Largest Sum Subarray Problem and I have used {3, -2, 5, -1, 4, -6, 2, 1} this array and find largest sum subarray is 9.
Index | Element | ‘max_current’ Calculation | ‘max_current’ | ‘max_global’ Calculation | ‘max_global’ |
0 | 3 | – | 3 | – | 3 |
1 | -2 | max(-2, 3 + (-2)) = max(-2, 1) | 1 | max(3, 1) | 3 |
2 | 5 | max(5, 1 + 5) = max(5, 6) | 6 | max(3, 6) | 6 |
3 | -1 | max(-1, 6 + (-1)) = max(-1, 5) | 5 | max(6, 5) | 6 |
4 | 4 | max(4, 5 + 4) = max(4, 9) | 9 | max(6, 9) | 9 |
5 | -6 | max(-6, 9 + (-6)) = max(-6, 3) | 3 | max(9, 3) | 9 |
6 | 2 | max(2, 3 + 2) = max(2, 5) | 5 | max(9, 5) | 9 |
7 | 1 | max(1, 5 + 1) = max(1, 6) | 6 | max(9, 6) | 9 |
Implement Kadane’s Algorithm in Python
Here’s a Python implementation of Kadane’s Algorithm
def find_largest_sum_subarray(arr):
max_so_far = arr[0]
max_ending_here = arr[0]
for num in arr[1:]:
max_ending_here = max(num, max_ending_here + num)
max_so_far = max(max_so_far, max_ending_here)
return max_so_far
# Example usage
array = [-2, 1, -3, 4, -1, 2, 1, -5, 4]
print("The largest sum of a subarray is:", find_largest_sum_subarray(array))
Here’s a C++ implementation of Kadane’s Algorithm
#include <iostream>
#include <vector>
#include <algorithm> // for std::max
int find_largest_sum_subarray(const std::vector<int>& arr) {
int max_so_far = arr[0];
int max_ending_here = arr[0];
for (size_t i = 1; i < arr.size(); ++i) {
max_ending_here = std::max(arr[i], max_ending_here + arr[i]);
max_so_far = std::max(max_so_far, max_ending_here);
}
return max_so_far;
}
int main() {
std::vector<int> array = {-2, 1, -3, 4, -1, 2, 1, -5, 4};
std::cout << "The largest sum of a subarray is: " << find_largest_sum_subarray(array) << std::endl;
return 0;
}
Detailed Explanation of Kadane’s Algorithm
Consider an array representing the temperature changes over a week and We need to find the period with the highest cumulative temperature change.
int arr[] = {3, -2, 5, -1, 4, -6, 2, 1};
Step 1:
First, we need to initialize two variables, max_so_far and max_ending_here, to the first element of the array. This sets our starting point for both the overall maximum sum we’ve found so far and the maximum sum of the subarray ending at the current position.
max_so_far = arr[0]; // 3
max_ending_here = arr[0]; // 3
Step 2:
Next, we iterate through the array starting from the second element. For each element, we update max_ending_here to be the maximum of the current element alone or the current element plus the previous max_ending_here. This checks whether to start a new subarray or continue the existing one. Then, we update max_so_far to be the maximum of itself and max_ending_here, ensuring we always have the highest sum found. below step represent each iterations values how Kadane’s Algorithm works.
- Current Day Change: -2
max_ending_here = max(-2, 3 + (-2)) = 1
max_so_far = max(3, 1) = 3 - Current Day Change: 5
max_ending_here = max(5, 1 + 5) = 6
max_so_far = max(3, 6) = 6 - Current Day Change: -1
max_ending_here = max(-1, 6 + (-1)) = 5
max_so_far = max(6, 5) = 6
Continue for Remaining Days…
By the time we’ve processed all elements, max_so_far holds the maximum sum of any subarray within the array. This final value gives us the answer to our problem, representing the highest possible sum we can get from a contiguous subarray.
Summarizing Kadane’s Algorithm in C++ and Python
Using Kadane’s Algorithm is a straightforward and efficient way to find the largest sum of a continuous subarray. By maintaining a running tally of the current subarray sum and updating the maximum sum encountered, the algorithm ensures an optimal solution in linear time. This approach is widely applicable in various fields, including finance, data analysis, and more, where locating maximum sum subarrays is essential.
By following these steps, you can effectively explain and demonstrate Kadane’s Algorithm concepts in C++ and Python. If you have any questions or need further clarification, don’t hesitate to ask on our Quora space or use the form on our website. Good luck with your interviews!
FAQs
What is Kadane’s Algorithm and why is it used?
Kadane’s Algorithm is a dynamic programming technique used to find the maximum sum of a contiguous subarray within a one-dimensional numeric array. It’s favoured because it works in linear time, making it highly efficient for large datasets. If you want to learn more about the logic behind Kadane’s Algorithm, check out our detailed explanation.
How does Kadane’s Algorithm handle negative numbers in the array?
Kadane’s Algorithm efficiently handles negative numbers by comparing the current element alone to the current element added to the previous subarray sum. This helps decide whether to start a new subarray or continue the existing one. For an in-depth understanding, visit our step-by-step guide on Kadane’s Algorithm.
Can Kadane’s Algorithm be implemented in Python?
Yes, Kadane’s Algorithm can be implemented in Python. The implementation involves iterating through the array and updating variables to track the current subarray sum and the maximum sum found so far. You can see an example of the Python implementation in our guide.
Why is Kadane’s Algorithm considered a greedy algorithm?
Kadane’s Algorithm is considered greedy because at each step, it makes a locally optimal choice to either continue with the current subarray or start a new one, aiming to find the global optimum. For more on this, explore our section on why Kadane’s Algorithm works.
What is the initial step in Kadane’s Algorithm?
The initial step involves setting two variables, max_so_far and max_ending_here, to the first element of the array. This establishes the starting point for tracking the maximum sums. For a practical example, check out our detailed guide.
How do you iterate through the array in Kadane’s Algorithm?
In Kadane’s Algorithm, you iterate through the array from the second element onward, updating max_ending_here and max_so_far based on the current element and the previous subarray sum. See how this works in detail in our algorithm breakdown.
How does Kadane’s Algorithm ensure it finds the largest sum?
By continuously updating max_ending_here and max_so_far as it iterates through the array, Kadane’s Algorithm ensures it captures the highest possible sum of any subarray. For a detailed explanation, refer to our guide.
Can Kadane’s Algorithm be implemented in C++?
Yes, Kadane’s Algorithm can be efficiently implemented in C++. The process involves using similar logic to the Python implementation but in a C++ syntax. You can see a full implementation example in our C++ guide.
What is a subarray in the context of Kadane’s Algorithm?
A subarray is a contiguous part of an array, meaning all its elements are consecutive within the original array. Understanding subarrays is crucial for applying Kadane’s Algorithm. For more examples and explanations, visit our subarray guide.