I recently came across a post about Inheritance in OOP on the AmbitionBox platform. I was shocked by this post because top Indian companies such as Wipro, Infosys, and TCS asks questions like ‘What is Inheritance in Object-Oriented Programming?‘, ‘Can you provide a real-life example of inheritance?‘, and ‘What are the different kinds of inheritance?‘ or ‘Types of inheritance in OOP?’ in their interview. Therefore, I have decided to cover the concept of Inheritance as a pillar of OOP in this blog post, where you will find answers to the aforementioned questions along with tips for your next coding interview.
Welcome back, interview preparers! How is your interview preparation going? I hope it is going well. In this blog post, I am going to share a complete guide on Inheritance in Object-Oriented Programming. By the end of this guide, you will be equipped to tackle this interview question successfully. Stay with me and enhance your skills for your next coding interview.
FREE : If you’re a new visitor preparing for an interview and need help or want to request a new question, you can use the form on the right side to ask your question. It’s free and doesn’t require signing in. Alternatively, you can ask in our Quora space, which is also free, and you’ll receive a response within 24 hours. Go ahead and check it out! do check Master the Stock Span Problem by 3 Easy Steps in Python, Master Abstract Factory Design Pattern for Programming Interviews with 5 easy steps ,Mastering Object-Oriented Programming in C++ , Method Overloading vs. Method Overriding in C#
Table of Contents
- Introduction to Inheritance in Object Oriented Programming
- What is Inheritance in OOPs ?
- Real Life Example of Inheritance in OOPs
- Types of Inheritance in OOPs
- Why Inheritance Matters in Programming Interviews
- Real-Life Development Example of Inheritance in OOPs
- Summarizing Ultimate Guide of Inheritance in OOPs
Introduction to Inheritance in Object Oriented Programming
I assume you are familiar with Object-Oriented Programming (OOP) and the benefits of adhering to its concepts. If not, allow me to provide a brief introduction to OOP. In simple terms, I would describe OOP as a method for writing minimalist, scalable, and well-maintained code. OOP is built upon four pillars: Abstraction, Inheritance, Encapsulation, and Polymorphism, which provide a concise overview of its principles. If you would like to learn more, please check out my post titled ‘Understanding Object-Oriented Programming (OOP) in C++.’
What is Inheritance in OOPs ?
Inheritance allows one class (child class) to acquire properties and methods from another class (parent class). This feature promotes code reuse, meaning you don’t need to rewrite existing functionality.
It’s important to understand that technical definitions may not be well-received in interviews. If you rely solely on precise definitions, the interviewer may assume you are using AI and could reject you.
In simpler terms, you can explain that inheritance addresses problems such as when we have a blueprint class and want to create another class based on that blueprint while adding further details. In such cases, we use inheritance to simplify code and enhance scalability.
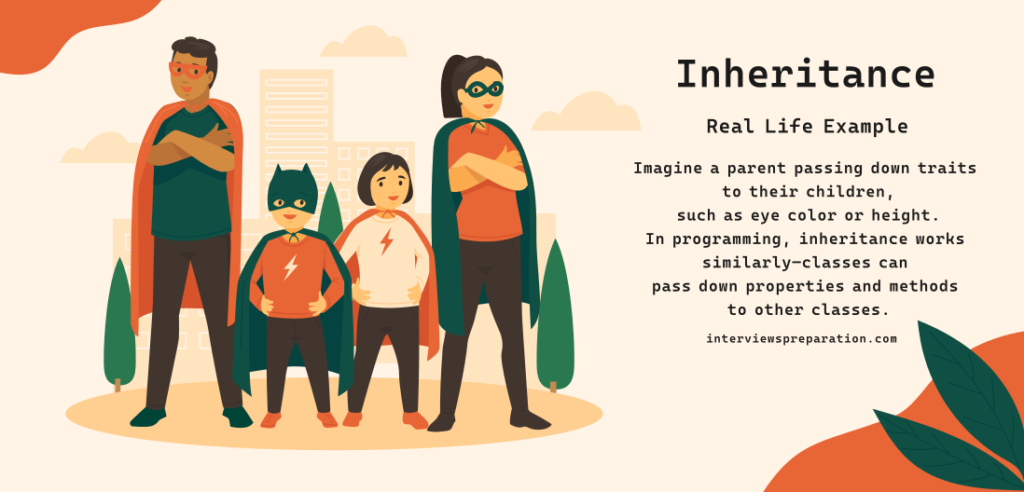
If you still find yourself confused about what to say, don’t worry! In the next section, I will provide real-world examples of inheritance in OOP. Interviewers often ask for this type of explanation as well.
Real Life Example of Inheritance in OOPs
Imagine a parent passing down traits to their children, such as eye color or height. In programming, inheritance works similarly—classes can pass down properties and methods to other classes.
class Parent {
public:
void speak() {
cout << "I can speak!";
}
};
class Child : public Parent {
// Inherits the 'speak' method from Parent
};
In this example, Child inherits the speak() method from Parent, meaning you don’t have to write it again in the Child class.
Types of Inheritance in OOPs
I will use C++ as an example to explain the types of inheritance because C++ has five inheritance types, allowing you to learn more about inheritance.
C++ supports five types of inheritance. Let’s explore each one with examples to see how they are used in different scenarios.
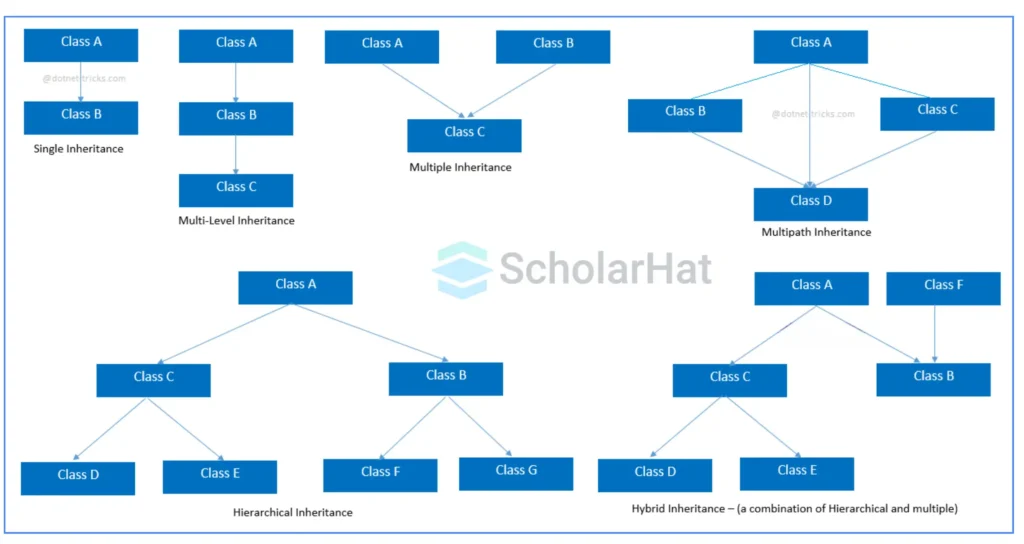
Single Inheritance in OOPs
In single inheritance, a class inherits from a single base class. Let us understand single inheritance with a real-world example.
Assume we have a class called Animal. Can you think of a common behaviour that all animals share? Please comment your thoughts. My answer is ‘to eat’. Therefore, if we want to create a Dog class, we can use Animal as the parent class to utilise the same method for the dog. Understood? Now, let us implement single inheritance in C++ code.
class Animal {
public:
void eat() {
cout << "Eating...";
}
};
class Dog : public Animal {
// Dog inherits the eat method from Animal
};
Multiple Inheritance in OOPs
A class inherits from more than one base class, which is known as multiple inheritance. Let us understand multiple inheritance in object-oriented programming with a real-life example. Let us consider animals again. Could you please provide a list of animals that can live both in water and on land? Comment your list if you have one. I will go with the crocodile, as this animal can thrive in both scenarios, correct?
Now, let us implement multiple inheritance in C++. As I described above, we have scenarios such as water and land; therefore, let us create a class named WaterAnimal and another named LandAnimal, both of which will have methods swim and walk.
The next step is to create a third class named Crocodile, which will implement both WaterAnimal and LandAnimal, as the crocodile can both walk and swim. I hope you are starting to grasp the concept of what is happening.
class LandAnimal {
public:
void walk() {
cout << "Walking on land...";
}
};
class WaterAnimal {
public:
void swim() {
cout << "Swimming...";
}
};
class Crocodile : public LandAnimal, public WaterAnimal {
// Crocodile inherits both walk() and swim()
};
Multilevel Inheritance in OOPs
In multilevel inheritance, a class is derived from another derived class. Let us explore this concept with a real life example.
We can begin with a base class called Organism, which has a method to represent breathing Next, we create a derived class called Mammal, which inherits the breathe() method from the Organism class.
Finally, we derive another class called Human from Mammal, allowing it to inherit the breathe() method through Mammal
class Organism {
public:
void breathe() {
cout << "Breathing...";
}
};
class Mammal : public Organism {
// Mammal inherits breathe() from Organism
};
class Human : public Mammal {
// Human inherits breathe() from Mammal
};
This structure demonstrates multilevel inheritance, where Human inherits the behaviour of breathe() from both Mammal and Organism.
Hierarchical Inheritance in OOPs
In hierarchical inheritance, a single base class is inherited by multiple derived classes. this is the most common inheritance we use in any programming language. let’s implement hierarchical inheritance using real live example.
class Vehicle {
public:
void start() {
cout << "Vehicle started.";
}
};
class Car : public Vehicle {
// Inherits start() from Vehicle
};
class Bike : public Vehicle {
// Inherits start() from Vehicle
};
In this example, we have a base class called Vehicle that contains a method start(). Both Car and Bike are derived classes that inherit from Vehicle, allowing them to utilise the start() method. This demonstrates how multiple derived classes can share common functionality from a single base class.
Hybrid Inheritance in OOPs
Hybrid inheritance combines two or more types of inheritance, incorporating aspects of multiple inheritance and hierarchical inheritance. let’s implement hybrid inheritance with real life example.
class Vehicle {
public:
void start() {
cout << "Vehicle started.";
}
};
class Car : public Vehicle {
// Inherits start() from Vehicle
};
class Boat : public Vehicle {
// Inherits start() from Vehicle
};
class AmphibiousVehicle : public Car, public Boat {
// Inherits from both Car and Boat
};
In this example, we have a base class called Vehicle with a method start(). The classes Car and Boat both derive from Vehicle, demonstrating hierarchical inheritance. Additionally, we have an AmphibiousVehicle class that inherits from both Car and Boat, illustrating hybrid inheritance.
This setup allows the AmphibiousVehicle to utilise methods and properties from both its parent classes while also inheriting the common functionality from Vehicle.
Visibility Modes in Inheritance: Public, Private, and Protected will cover in next post.
Why Inheritance Matters in Programming Interviews
Understanding and implementing inheritance demonstrates to interviewers that you can structure your code efficiently. Here are some key points to remember:
- Code Reusability: Inheritance allows you to reuse existing code, reducing redundancy.
- Readability & Maintenance: Inheritance makes your code more organized, which helps with long-term maintenance.
- Abstraction & Polymorphism: It promotes abstraction by hiding complexity, and it supports polymorphism, allowing one interface to control multiple behaviors.
Real-Life Development Example of Inheritance in OOPs
Let’s say you’re developing a game where different characters have shared abilities like jumping or running. Instead of defining these abilities for each character, you can create a base class for Character and inherit common abilities.
Please ensure that your real-life development example encompasses all aspects of inheritance; otherwise, the interviewer may perceive it as a lack of knowledge. Also, remember that this is one of the most common questions asked in object-oriented programming interviews at leading tech companies in India.
class Character {
public:
void jump() {
cout << "Jumping!";
}
void run() {
cout << "Running!";
}
};
class Warrior : public Character {
// Inherits jump and run from Character
};
class Mage : public Character {
// Inherits jump and run from Character
};
Both Warrior and Mage inherit the jump and run methods from the Character base class, keeping the code DRY (Don’t Repeat Yourself).
Summarizing Ultimate Guide of Inheritance in OOPs
Inheritance in C++ is not just an OOP concept but a powerful tool for simplifying your code structure. By using inheritance effectively, you can make your programs more flexible, maintainable, and efficient—qualities that are sure to impress in your next coding interview!
Happy coding and best of luck with your interviews!